Welcome to Start with Swift! This is a new “series” to get to know the basics of the Swift programming language. Want to start programming? Swift is an ideal choice to start with because it is expressive and easy to understand.
Now let’s get started. In this article we will look at simple types. They are used in all programming language (sometimes with some syntax (“grammar”) modifications). Here belong the most basic, yet the most used commands.
Variables
Variables are used for storing data. The word itself says that values can vary –> they are mutable. It means they can change its value later. For using variables we use the shortcut “var”.
var str = “Hello, World!”
Var is a command, which creates a variable we named “str”. You can name a variable however you want, but shorter names are recommended because they’re easier to remember and quicker to type.
After a name we write “=“ symbol which then refers to the value. We can’t really say that it means “equals to” because equality is declared in a slightly different way (you’ll get to know later). I’d say it just means “is”.
We’ve set the value as “Hello, World!” which is a string. More about strings below!
If you want to change the value, all you need to do is:
str = “Goodbye!”
When you’re changing values, you don’t need to type the command “var”, because variable “str” already exists.
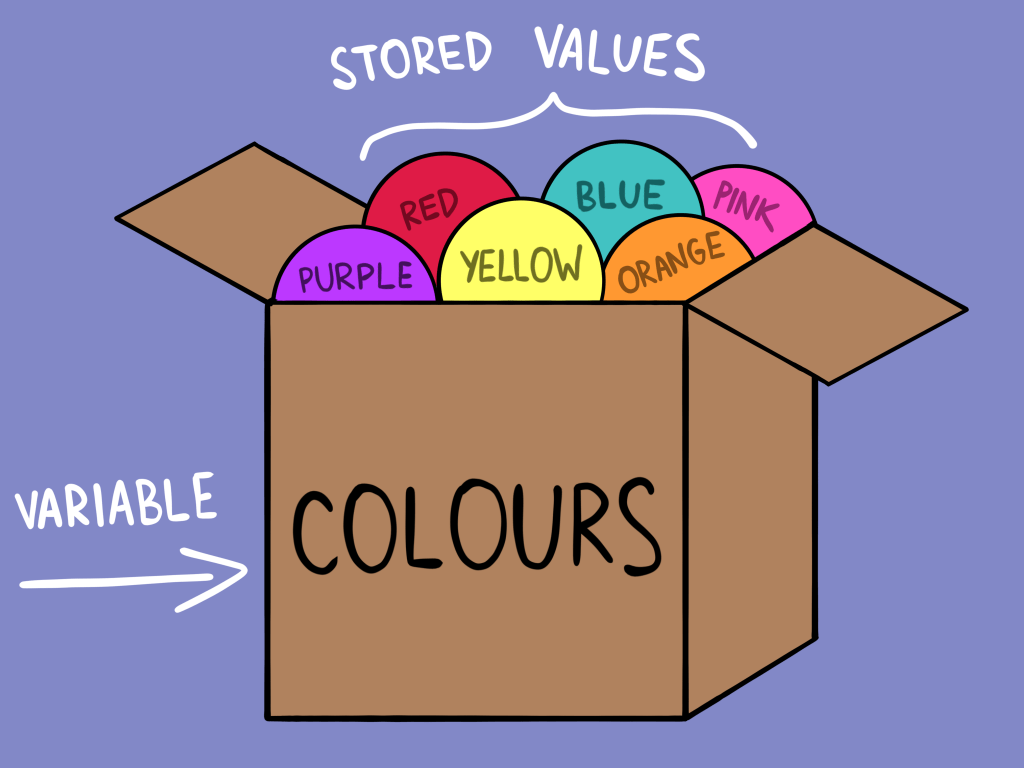
Strings and integers
As mentioned before “Hello, World!” and also “Goodbye!” are strings. We use strings for letters, words and expressions. They are written in apostrophes.
Whereas integers are used for and only whole numbers. So if you type for example:
var age = 23
It means you’ve created a variable with an integer value, which is the number 23. The type of the value is assigned as Int, which is the shortcut for the looong word integer.
If you want to use a bigger number you need to use underscores (_). They work as thousand separators. So you write for example 7 million as 7_000_000.
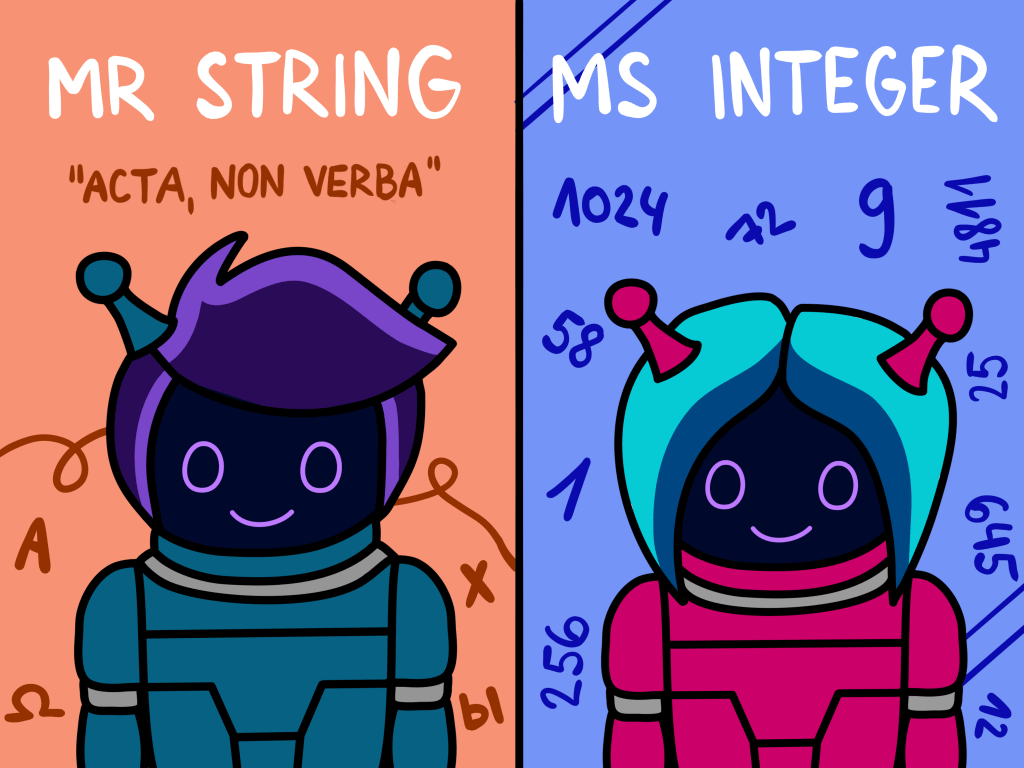
You cannot mix strings and integers. They are different types.
Doubles
But what if I want to use number 1,6? Doubles are made exactly for fractional numbers like 1,6! Remember, doubles can’t be mixed with integers, so in a nutshell: for whole numbers like 23 use integers and for fractional ones like 1,6 use doubles.
var fn = 1.6
Note: Some of us are more used to write a comma for these numbers, but for the “point” symbol, use a dot instead of a comma.
Booleans
Booleans are used to assign whether the value is true or false.
Constants
Constants are used basically for the same reason as variables: storing data. But they are different in one thing: constants are not mutable. They are used for setting values for once and never again. For using constants we use a command “let”.
let camilla = robot
A big question is when to use variables and when constants. It depends if you want or if it’s logically possible to change the value later. But at the beginning I would recommend to always use a constant. If you decide to change the value later, the program will warn you to replace “let” with “var”. That’s no big deal.